Understanding the Distinctions: map() vs flatMap() in Java Streams
Written on
Chapter 1: An Introduction to Java Streams
If you're a Java developer, whether experienced or just starting to explore functional programming, you've probably come across the map() and flatMap() methods. While they might appear similar at first glance, these methods are fundamental components of Java's Stream API and serve distinct functions.
Recognizing the differences between map() and flatMap() is not merely about syntax; it's about grasping the nuances that can enhance the efficiency and clarity of your code. In this section, we'll embark on an exploration of these functions, acknowledging their individual characteristics and learning when to apply each in your programming tasks.
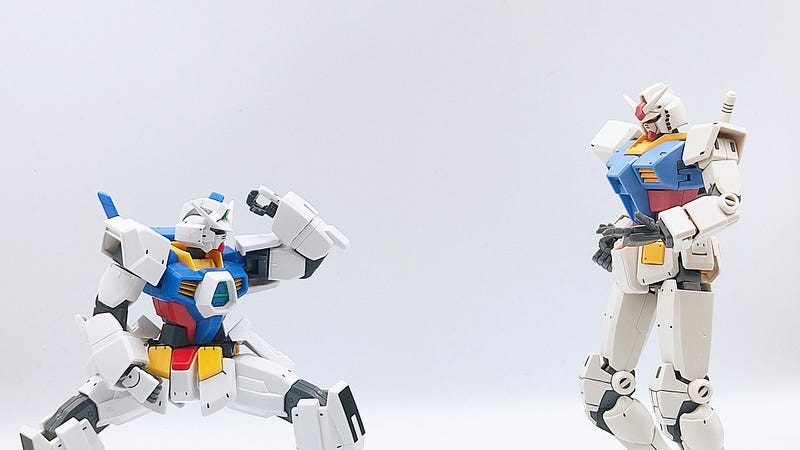
Photo by Jeffery Ho on Unsplash
Chapter 2: Stream API: A Brief Overview
Before we dive deeper into the specifics of map() and flatMap(), it’s worthwhile to briefly examine the Stream API—the framework within which these functions operate. Launched in Java 8, the Stream API transformed data processing in Java, enabling a more declarative programming style.
Think of streams as conduits for data, allowing a sequence of operations—like map, filter, and sort—to be performed in a functional manner. Unlike data structures, streams facilitate the application of transformations on underlying data sources such as collections, arrays, or input/output channels.
One of the standout features of the API is its capacity to manage vast amounts of data seamlessly, optionally harnessing parallel processing for enhanced efficiency. Utilizing streams allows you to articulate what you aim to achieve with the data, rather than how to achieve it, resulting in code that is both more readable and maintainable.
Chapter 3: Understanding the map() Method
The map() method in the Stream API allows you to apply a specified function to each element within a stream. It’s particularly useful for transforming data; for instance, you might use it to convert a list of strings into a list of their lengths, or to transform a stream of orders into their total prices.
Consider the following example:
List<String> words = List.of("Java", "Stream", "API");
List<Integer> wordLengths = words.stream()
.map(String::length)
.collect(Collectors.toList());
System.out.println(wordLengths); // Outputs: [4, 6, 3]
In this case, we have a list of words that we want to convert into their respective lengths. By applying the map() function, each string is transformed into its length, resulting in a new list.
Practical applications of map() often include straightforward, one-to-one transformations where each input element corresponds to exactly one output element, without concern for nested structures.
Chapter 4: Exploring the flatMap() Method
The flatMap() method is another transformative function within the Stream API. It first applies a function to each stream element like map(), but then it flattens the resulting elements into a new stream. The primary advantage of flatMap() is its capability to manage intricate, multi-layered data structures by merging them into a single layer.
A simple example will illustrate flatMap() in action:
List<List<String>> listOfLists = List.of(
List.of("Java", "Stream"),
List.of("flatMap", "method")
);
List<String> flattenedList = listOfLists.stream()
.flatMap(Collection::stream)
.collect(Collectors.toList());
System.out.println(flattenedList); // Outputs: [Java, Stream, flatMap, method]
In this example, we have a list of lists containing strings. The flatMap() method processes each list, streaming its elements and combining them into a single flow. The outcome is a flattened list of strings.
This method is particularly beneficial when dealing with nested data structures, such as lists of lists, where you want to consolidate all elements into one sequence—often necessary for further operations.
Chapter 5: Key Differences Between map() and flatMap()
Grasping the key differences between map() and flatMap() is essential for choosing the appropriate function for a specific task. Here are the main distinctions:
- Type of Operation: The map() function executes a one-to-one mapping, meaning each input element corresponds to a single output element. Conversely, flatMap() is utilized for one-to-many mappings, where each input element can yield multiple output elements that are then "flattened" into a singular stream.
- Return Types: The map() function produces a stream composed of the results from applying the specified function to the original stream elements. In contrast, flatMap() requires the provided function to yield a stream of elements, which it then concatenates into a single, flat stream.
- Handling Nested Structures: When working with streams that involve complex structures—like streams of streams or lists of lists—map() applies transformations only to the outer layer. In contrast, flatMap() flattens and merges these structures into a single level, making it the preferred option for deeper transformations.
To illustrate these differences, consider the following example:
List<String> words = List.of("Hello", "World");
List<String[]> mapResult = words.stream()
.map(word -> word.split(""))
.collect(Collectors.toList());
List<String> flatMapResult = words.stream()
.flatMap(word -> Arrays.stream(word.split("")))
.collect(Collectors.toList());
In this scenario, the map() method produces a list of string arrays (one array for each word, containing the individual letters), while flatMap() flattens all letter strings into a single list.
Given these points, developers should opt for map() when performing straightforward, per-element transformations and choose flatMap() for comprehensive transformation and flattening of nested structures.
Chapter 6: Conclusion
In conclusion, both map() and flatMap() are vital methods within the Stream API for Java developers. A solid understanding of these functions and their differences is essential for harnessing the full potential of functional programming in Java. Whether you're transforming a list of objects or flattening a complex structure, selecting the correct method can significantly improve the readability, maintainability, and performance of your code.
Thank you for your attention! Happy Coding!
Thank you for sticking around! If you found this content valuable, consider showing your support by clapping and following the author! Connect with us on X | LinkedIn | YouTube | Discord. Explore more content at Stackademic.com.